7 Steps to Master Coding: A Beginner's Guide
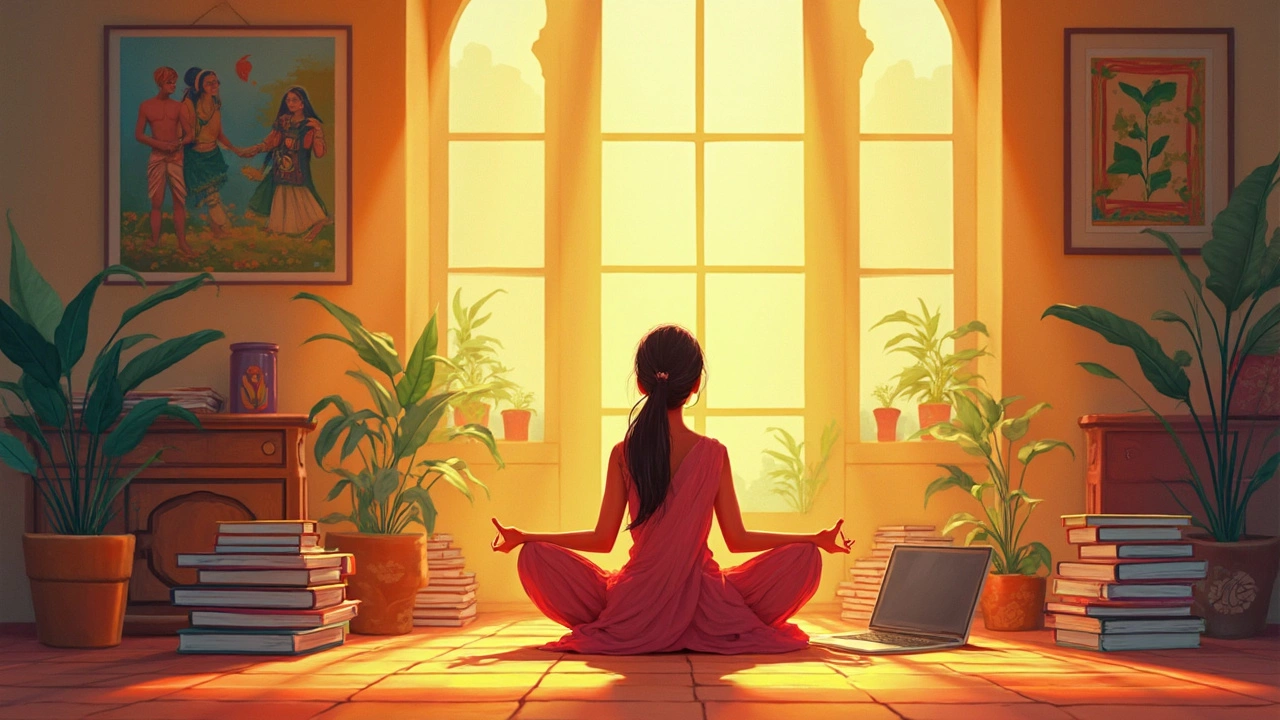
Diving into coding might feel like stepping into a new universe. But breaking it down into digestible tasks works wonders. Step one? Understand the problem. Before even touching the keyboard, make sure you really get what's being asked. If it’s a simple calculator app, ask yourself, what features do you want? Addition, subtraction, or more?
Next up, plan your approach. This step is all about brainstorming and decision-making. How are you going to tackle the problem? Sketch out a basic flow of how the program should work. Think of this as your game plan, helping you see the bigger picture.
- Understand the Problem
- Plan Your Approach
- Write Pseudocode
- Choose the Language
- Start Coding
- Debugging and Testing
- Optimize and Reflect
Understand the Problem
Before you dive into any coding, the most critical step is to fully grasp what you're trying to solve. Skipping this step is a recipe for frustration and wasted effort, no matter how skilled you become at writing code.
What's the Task?
First off, break down the task at hand. Is it a simple program like a counter or are you dealing with something more complex, like a web application with a database? Knowing your task inside and out is crucial.
Ask the Right Questions
Get in the habit of asking questions. If you're working on a beginner programming project, ask yourself: Who's using this? What does it need to accomplish? Are there any constraints like time limits or specific technologies you have to use?
Break It Down
Once you have a good grasp of the problem, fragment it. Divide the big task into smaller, manageable pieces. For example, if your task is to build a blog, think about the essential features: user accounts, post submissions, and a comment system.
Create the Big Picture
Mapping out these components helps see how each piece fits together. It’s like solving a puzzle. This step sets you up for efficient coding and reduces chances of hitting a dead end.
If you're coding with a team, it's vital everyone agrees on the problem. Misunderstandings at this stage can become massive roadblocks later.
Plan Your Approach
Jumping straight into coding can lead to confusion and time wasted on errors. Taking a moment to plan your approach is a game-changer. It’s like creating a roadmap that guides you from start to finish. Focusing on this step sets a strong foundation for coding success.
Break Down the Problem
Start by breaking the problem into smaller, manageable parts. This technique, known as 'divide and conquer,' simplifies complex problems. Imagine building a house; you wouldn’t start by decorating, right? Think about what the key parts are and tackle them one by one.
Design Your Solution
Think of this as sketching a blueprint. You want to outline the logic of your program without writing any actual code. Consider flowcharts or diagrams if visual tools help you conceptualize. This step clarifies what the program needs to do and reduces the potential for errors later.
Think Reusability
Reusability is a lifesaver. While planning, think of parts of the code you can reuse, not just in this project but in future ones. Functions and libraries that can be repurposed save tons of work down the track. This is a crucial part of efficient coding.
Set Priorities
Prioritizing tasks makes tackling them less overwhelming, especially when dealing with large projects. Identify which parts of the program are critical and should be completed first. Knowing your priorities keeps you focused and productive.
Map Out a Timeline
Don’t forget the timeline! Decide how much time to allocate to each step in the process. This helps maintain steady progress without the pressure of impending deadlines. Plus, it keeps you accountable and on track.
Write Pseudocode
Once you've got your plan laid out, it’s time to write pseudocode. Sounds fancy, but it's just regular language describing what your code will do. It’s like a recipe for your program. It doesn't matter what programming language you're using; pseudocode is all about logic and flow.
Why Write Pseudocode?
Pseudocode is a lifesaver, especially for beginners. It helps you think logically without getting tangled in syntax errors. According to MIT's Programming Course, "Pseudocode lets you focus on the problem rather than the coding language."
Think of pseudocode as your first draft. It lets you map out each step your program will take. Many expert developers swear by it because it minimizes mistakes when you finally write real code, saving time on debugging later.
How to Write Pseudocode
- Start by breaking down your problem into smaller, manageable tasks.
- Write each task as a simple statement or instruction.
- Use plain English and keep it clear and precise.
- Focus on the logic and order of operations.
For example, if you’re coding a simple app to calculate the sum of two numbers, your pseudocode might look like this:
- Input the first number.
- Input the second number.
- Add the two numbers.
- Display the result.
It’s simple, right? But here's the magic: when you start coding, you’ll know exactly what needs to go where, and that’s worth its weight in gold.
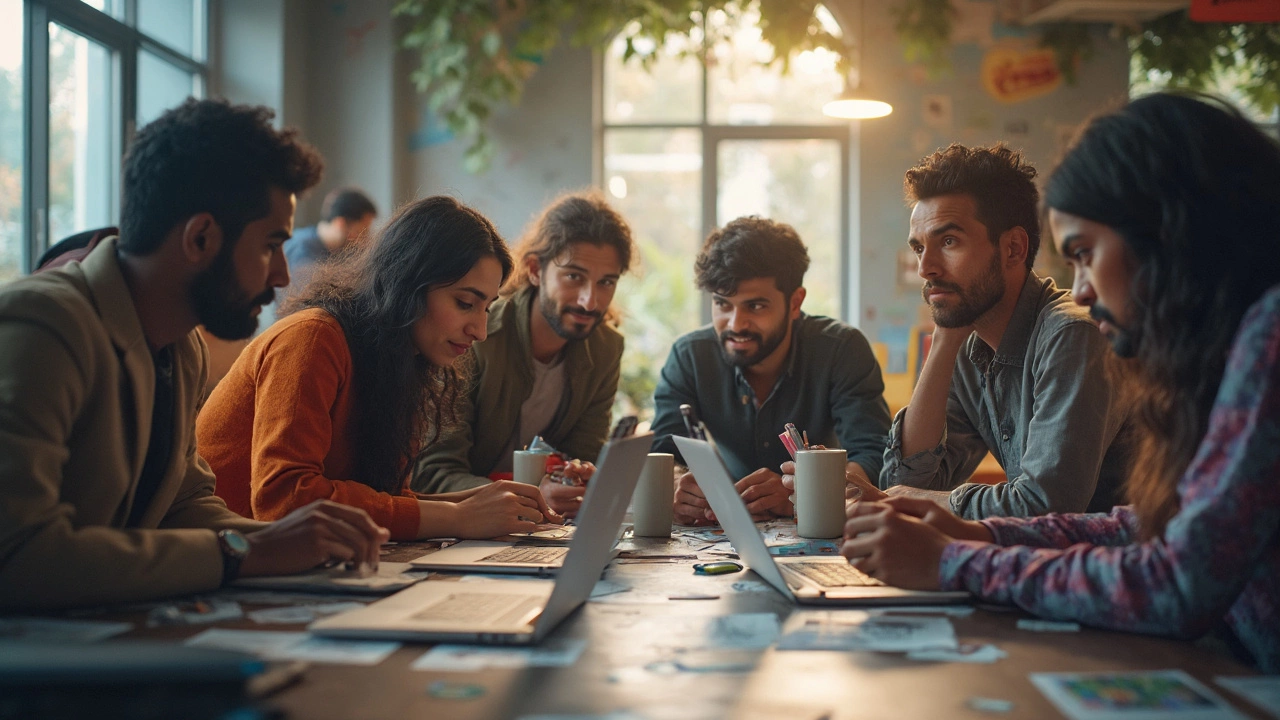
Choose the Language
This step is where many start getting pumped—picking your programming language. The landscape is vast, with numerous languages having unique perks. But the big question is, which one should you start with? The truth is, it depends on what you're aiming to achieve.
Python: The Beginner's Delight
Python is a favorite for beginners due to its robust simplicity. It has a clean and easy-to-read syntax that feels almost like English. Want to dive into web development, software engineering, or even data science? Python's got you covered.
JavaScript: The Web's Bestie
If you're eyeing web development, JavaScript should be your go-to. It's the backbone of web applications and lets you create dynamic content. Think about those eye-popping animations or interactive site elements—that's JavaScript at play.
Java: Stay Strong with Stability
Java is another solid choice, especially if you're interested in mobile apps or enterprise-level applications. Many big corporations rely on Java for their backend systems, so learning it can open up a lot of coding doors.
When picking a language, remember there's no 'right' choice. Consider your goals and which language aligns with what you want to build. Some folks even suggest dabbling in a couple of languages to find which suits your thinking process best. Whichever path you choose, sticking with it is key. Mastering one language thoroughly often makes learning others quicker and easier.
Start Coding
So, you've got your game plan ready. It’s time to jump into the actual fun part: coding. Starting can feel overwhelming, but it's really the part where you bring your ideas to life. This is where your plans turn into digital reality. It's important to remember that your first draft of code is just that— a draft. Don't worry about getting everything perfect right away.
Begin by setting up your coding environment. Choose an Integrated Development Environment (IDE) or a simple text editor that suits your preferences. If you're starting with languages like Python, VSCode or PyCharm could be your go-to. For web development, HTML, CSS, and JavaScript can be coded directly in editors like Sublime Text or Atom.
Write Basic Structure
Every line of code you write matters, but start simple. Create the skeleton of your program first—something that completes the basic objectives. Don’t get caught up in the nitty-gritty right from the start.
Follow Best Practices
Consistency is key in actual programming. Choose a naming convention for your variables, like camelCase or snake_case, and stick with it. This helps keep your code organized and readable.
- Comment Your Code—It might seem tedious, but comments are lifesavers for you and anyone else reading your code.
- Save Regularly—Be the person who doesn't lose hours of work due to a power failure.
- Use Version Control—If you're serious about coding, learning Git is a smart move. It keeps your project backed up and allows easy collaboration if you're working with a team.
Finally, don't shy away from using resources available online. Sites like Stack Overflow and GitHub are treasure troves of free information, providing answers to almost any coding question you might have. Coding is not about memorizing everything—it's about knowing where to find the answers.
Debugging and Testing
Here's the truth about coding: errors are going to happen. It's pretty much inevitable. That's where debugging steps in, the process of fixing those pesky mistakes. Think of it as your program's health check.
First things first, run your code to see if it works. If it crashes—or gives you unexpected results—fear not. This is where things get interesting. Debugging usually starts with keyword-rich strategies. Use tools like breakpoints or console.log() to find where your code is going wrong. It's like a detective hunt, finding where things aren’t going as planned.
Common Tools and Techniques
Integrated Development Environments (IDEs) like Visual Studio Code or PyCharm have built-in tools to track these errors. They let you step through code and watch variables change in real-time. Don't forget about online resources too; forums like Stack Overflow are lifesavers when you're stuck.
The Importance of Testing
Once your code is error-free, it’s time for testing. Make sure your program does what it’s supposed to by putting it through its paces. Create various 'test cases' – different scenarios to check every corner of your code behaves properly.
- Unit Testing: Checks if small parts of your program (like functions) work well.
- Integration Testing: Ensures combined parts all function together.
- User Acceptance Testing: Ultimately, it's about your software being user-ready. Would your grandma be able to use it without calling you five times?
In the end, successful debugging and testing ensure that you've not just written code, but built a reliable piece of software. It’s all about squashing those bugs, tweaking that logic, and making it as smooth as your favorite playlist.
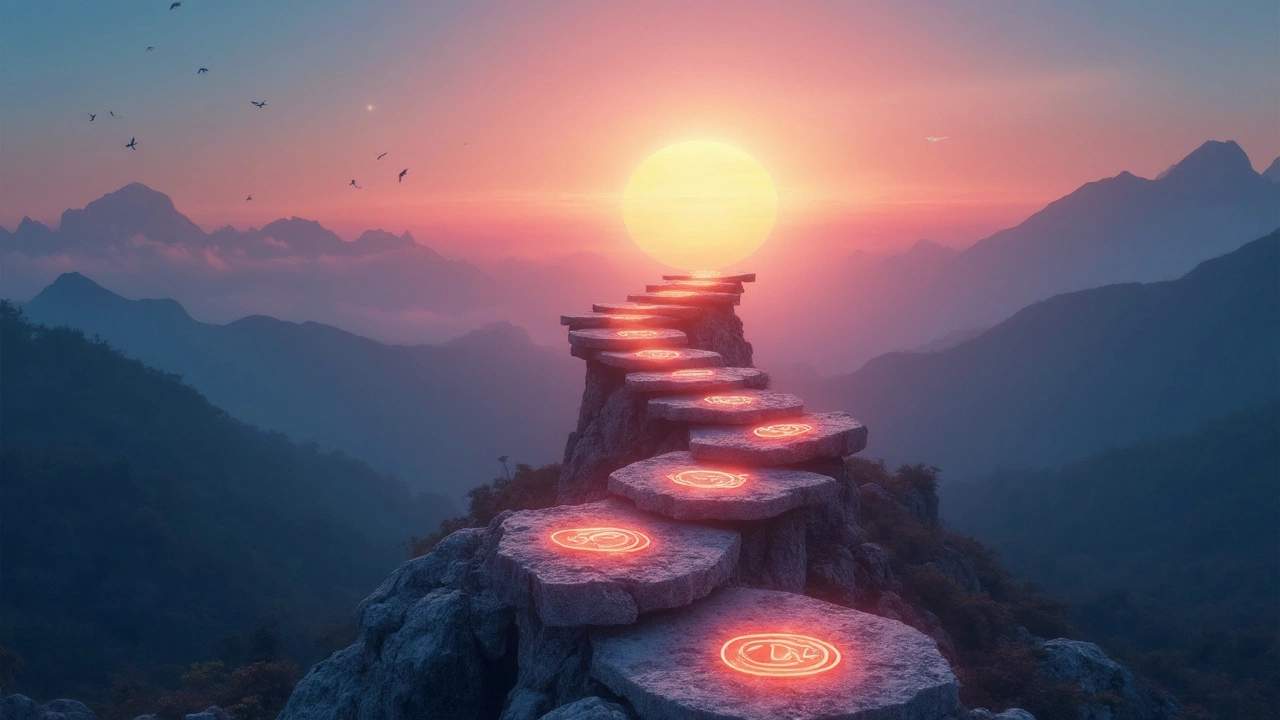
Optimize and Reflect
Once you've got a working piece of code, you might think you're done. But hold up! The real magic happens in optimizing and reflecting on what you've built. This isn't just about making your code faster—though that's pretty crucial, too. It's about making it more efficient and simpler to read. It's not uncommon to reduce code length by 20% through optimization.
Start with simplifying your code. Look for repetitive lines that can be combined. If you're using Python, see if there are built-in functions or libraries that can make your life easier. Remember, readable code is as important as functional code, especially when you're working in a team.
Performance Check
Are there bits of your code that slow everything down? Use debugging tools to trace performance and find bottlenecks. Maybe a certain function call is eating up resources. Use performance profiling tools to check this out.
Reflect and Document
This step is often overlooked, but reflection is an essential part of coding. Document what you’ve learned: what went well and what didn’t? Jot down your journey in comments within your code. This not only helps you but also others who might look at your coding steps in the future.
Optimization isn't just for your computer—it's for your skills, too. Each project is a building block in your learning path. Take these reflections with you as you move on to your next coding adventure.